User Guide¶
Installation¶
Install Imaging Source software IC to verify that cameras are accessible and working corectly.
Select current driver for Scorpion.
The scorpion camera driver requires tis_udshl12.dll present in the path.
Camera Setup¶
Camera properties can be set by using configuration dialog or via Python interface
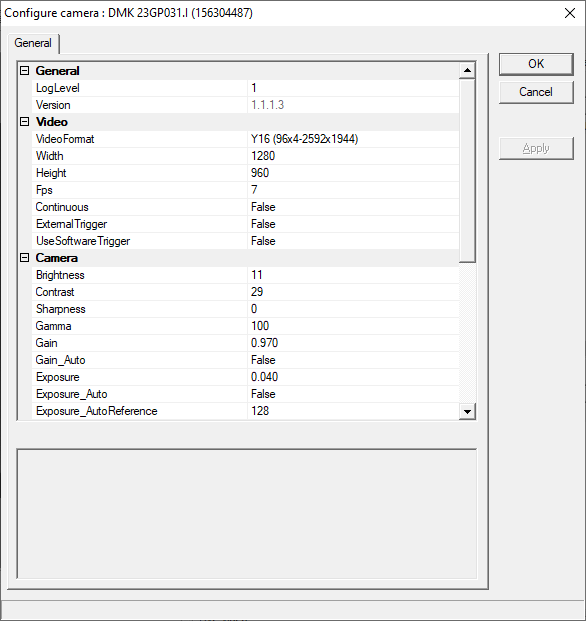
Change one or several properties. Watch notifications at the very bottom of the dialog.
When one or several property are changed Apply button gets enabled.
Pressing Apply writes properties to camera and then reads back them again. It is a convenient way to determine if given property is accepted by the camera. Or if changing one property changes others.
Read-Only values will appear grayed.
Note
Video Format, Width, Height can only take effect after closing/reopening the camera: set the values, close the dialog by pressing OK and then reopen dialog again.
Setting properties via Python¶
All camera properties can be accessed from Python.
An example below provides possible use cases:
cam= GetCamera("0")
#set new width to 1600
cam.executeCmd('set', 'Width=1600')
#find out image height
print 'height=', cam.executeCmd('get', 'Height')
# toggle hw trigger on
cam.executeCmd('set','ExternalTrigger=true') # enables trigger mod
# disable hw trigger
cam.executeCmd('set','ExternalTrigger=0') # Or 'ExternalTrigger=False'
Property name can be obtained from configuration dialog.
Note
Camera must not be in live grabbing mode. Not all properties can be accepted if camera is in live mode.
Some properties are available exclusively via python script. That includes the ‘button’ properties.
Property | Type | Description |
---|---|---|
LogDebugView | get/set | debug level, [0..3]. Outputs diagnostics to DebugView application. |
continous | get/set | boolean (1 or 0), video stream continuous mode, passing all frames to Scorpion regardless of grab command |
SoftwareTrigger | set | execute software trigger, if available |
a button property | set | some properties are ‘buttons’ as they are called. Setting their value to 1 will execute that command |
For example:
cam= GetCamera("0")
#issue a software trigger
cam.executeCmd('set', 'SoftwareTrigger=1')
Grabbing images via Python¶
Normally a Grab command from Scorpion will produce a single image. That creates a significant overhead when high fps is involved, because camera enters a live mode, grabs an image, and then leaves the live mode. Unnecessarily, because right after that it will have to enter live mode again.
Note
Use continous mode for the fastest grabbing.
How to start continous mode:
cam= GetCamera("0")
# enter continous mode and start grabbing according to the current fps. No Grab commands needed.
cam.setProperty('continous', 1)
# end continous grabbing
cam.setProperty('continous', 0)
Grab N images in continous mode:
#This allows to grab specified number of images and stop automatically.
N= 50
cam.setProperty ('continous', N)
#No command needed to stop continous mode
Setting properties using specialized Python class¶
Use CameraProperties class from CameraProperties.py to transfer setting of parameters into Scorpion scripts.
The advantage is fine tuning of the parameters, having several sets of them, and ensuring that camera gets required setup everytime profile starts.
CameraProperties class contains named definition of cameras (or camera types). Each definition contains complete set of properties.
Currently defined cameras:
- ImagingSource23GP031
Class functions¶
CameraProperties cam(0,'ImagingSource23GP031')
#get list all defined named property sets
cam.GetCameras()
#get list of defined properties for the particular camera
cam.GetProperties()
#get type of the property. It can be one of ['enum', 'int', 'float', 'bool']
cam.GetType(prop)
#get defined range of the property, or enumeration, as a list.
#bool type will return empty list
cam.GetRange(prop)
#get list of properties that are 'buttons'
cam.GetButtons(self)
#convienently print all of the parameters into console
# ready to be pasted into another script and edited as necessary
cam.PrintStub(indent=4)
#set property
cam.SetProp(name, value)
Usage examples¶
import CameraProperties
#Create the object.
CameraProperties cam(0, #camera 0, as in GetCamera('0')
'ImagingSource23GP031', #named camera property set
2) #the highest verbose level. Default is 1
#set some parameters
cam.SetProp('fps', 25)
cam.SetProp('Contrast', 10)
Usage tips¶
To avoid hand-writing all parameters into a script the following procedure can be invoked
- Create temporary script for stub generation:
from CameraProperties import CameraProperties
CameraProperties cam(0,'ImagingSource23GP031')
cam.PrintStub(2) #2 is indent. Default is 4
- Open the Scorpion console. Make sure camera messages were enabled prior to Step 1. Find the generated text, it should look similar to:
cam=CameraProperties(0,'ImagingSource23GP031',1)
cam.SetProp('fps', '25') # enum [2.11, 3, 4, 5, 6, 7, 8, 9, 10, 12, 14, 16, 18, 20, 25, 30, 35, 36.3]
cam.SetProp('Brightness', 100) # int [0, 4095]
cam.SetProp('Contrast', 10) # int [-10, 30]
cam.SetProp('Hue', 100) # int [-180, 180]
...
- Paste the code into the permanent script, modify script runtime settings. Edit camera properties as necessary.
Extending CameraProperties class¶
When extending CameraProperties make sure to append correctly new data to Preset, Buttons and Preset_orderedList structures.